As the use of R in the scientific community has grown, so has the demand for tools that can help scientists and researchers visualize their data. Plots such as bar plots, box plots, histograms, and others are useful for almost any analysis, but they don’t always fit easily into the language of mathematics. In this post, I will discuss the approach I’ve taken to creating functions in R to produce plots that are easy to use, and can also be customized via parameters.
Over the past few years, the popularity of R has skyrocketed. This is due to its ability to make quick and easy data visualizations, and the fact that it is free and open source. The language has evolved over the years, but is still relatively easy to learn. The real power of this language, however, comes from the package ggplot2 which allows for the creation of complex graphics. Here, we will learn how to use ggplot2 to create graphs using functions.
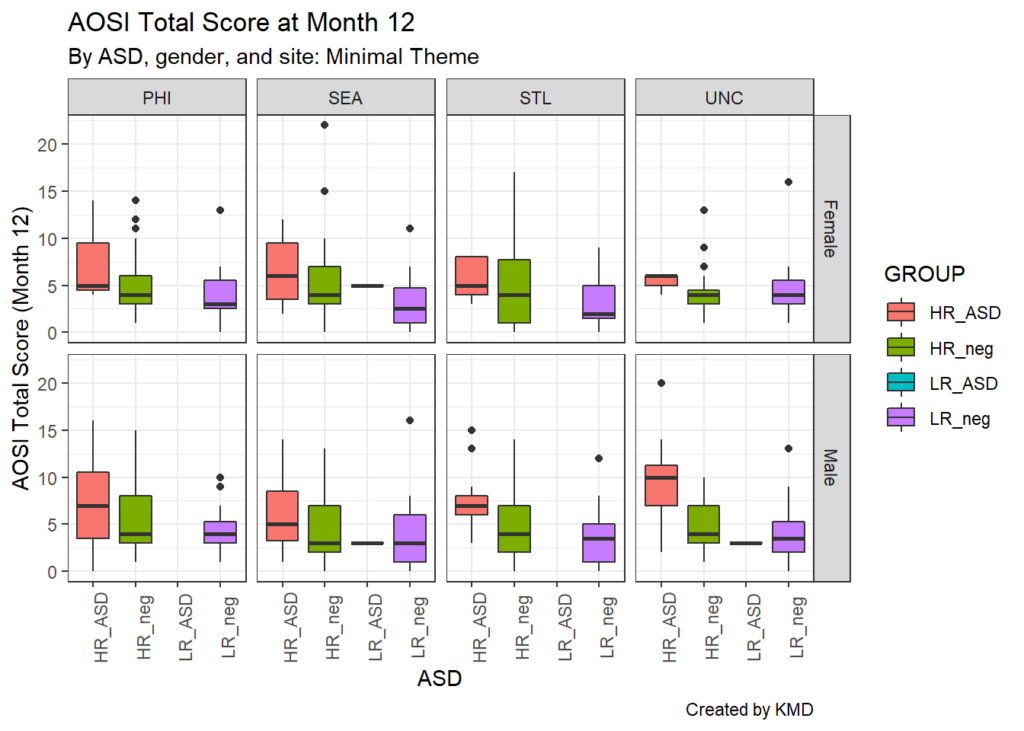
ggplot2 is a R package for making beautiful graphics, and it can do so even within the R studio Desktop environment. The package is intended to replace the base functions provided by R, which support the creation and publication of plots. The R package is a big improvement over the base functions because it reduces the amount of code and code dependencies in the plots.
Okay, here’s a confession. I often preach about writing editorials to make my life easier at work. While I try to follow the mantra of writing functions decently, there is a grey area where I don’t use that many functions. Do you have any ideas? Often tons of search graphs are created with ggplot2, with tons of code repetition with little change in variables. It has nothing to do with lack of effort. I’ve tried writing functions for plotting that use clean estimates (see the proof here).
However, this custom was never adopted. Here, I am again trying to write functions to make graphs. I came across two great resources: first, the RStudio 2024 Best practices for programming with ggplot2 report by Dewey Dunnington and a recent talk by Claus Wilke. I’ll start here with a simple example, courtesy of Klaus Wilke’s class on functions and functional programming. Here is an example of how to write functions to plot with ggplot2. library(tidyverse) library(palmerpenguins) Suppose you need to create a graph of data corresponding to a group, i.e. a subset of the data. In this example we use the Palmer’s penguin data and draw only one of the penguin species. Our natural approach is to filter the data according to the group of interest and display it in a graph. Penguins %>% filter(species == Gentoo) %>% ggplot() + aes(beak_length_mm, body_weight_g, color=sex) + geom_point() + ggtitle(species : Gentoo) + xlab(beak_length (mm) + ylab(body_weight (g)) + theme(plot.title.position = plot) Scatterplot with repeated batch code If you want to plot different groups, you can often repeat most of the code except for the part that indicates the group of interest. The different species of penguins are presented here. Note that the code is almost identical except for the filter operator and the ggtitle operator. Penguins %>% filter(species === Chinstrap) %>% ggplot() + aes(beak_length_mm, body_mass_g, color=sex) + geom_point() + ggtitle(species : Chinstrap) + xlab(beak_length (mm)) + ylab(body_mass (g)) + theme(plot.title.position = plot) Scatterplot with Many Code Repetitions Example 2 We can avoid writing similar code by using variables and functions. For example, instead of hard coding group values, we can create a variable and use a variable name instead.
For example, we can create a new variable to specify a subset of interest, in this case the type of interest. And use the name of the variable when plotting, that will help us not to write similar code. For example, we define a new species_choice variable with the desired species. Species_selection <- Adelie penguins %>% filter(Species == Species_selection) Another trick is to access the variable of interest from another environment. This allows us to use the same variable name in different environments. For example, we can specify the desired species by Species <- Adélie And a subset of data with the view name, but from two different environments. Here we use the pronoun .data to access the view variable from the data and .env to access the view variable from the current working environment. Penguins %>% filter(.data$species == .env$species)
Here, .data$species specifies the column in the data frame, and .env$species is the variable in the local environment we just created. We can now write a small function that takes the name of the input image and traces it. Note that we use the sticky packet trick to access the variable name by using braces around the variable in question. Glue provides interpreted strings that are small, fast, and independent of dependencies.
To do this, Glue encapsulates R expressions in braces, which are then evaluated and inserted into the argument string. make_plot <- function(species) { penguins %>% filter(.data$species == .env$species) %>% ggplot() + aes(spine_length_mm, body_mass_g, color=sex) + geom_point() + ggtitle(glue(Species: {species})) + xlab(spine_length (mm)) + ylab(body_mass (g)) + theme(plot.title.position = plot) } We can call a function to draw a view. make_plot(Adelie) With the diagram function, we can easily create a graph of all types without repetition. Here we use the map function, which takes each element of a view vector and uses it as input to make_plot(). And the resulting graphs are stored in a variable as a list. Species <- c(Adelie, Kinband, Gentoo) plots <- map(Species, make_plot) We can take plots off the list. We can obtain the first graph Lots[[1]] and the second floor. Lots[[2]]For many people, there is nothing more exciting than great data visualizations. While you can use R to do a lot of things, creating a plot with ggplot2 can be a daunting task. In this post, I will show you how to create some basic plots with ggplot2 in R using a simple function I wrote called plot_simple.. Read more about could not find function “ggplot” and let us know what you think.
Frequently Asked Questions
What are various plotting functions in ggplot2?
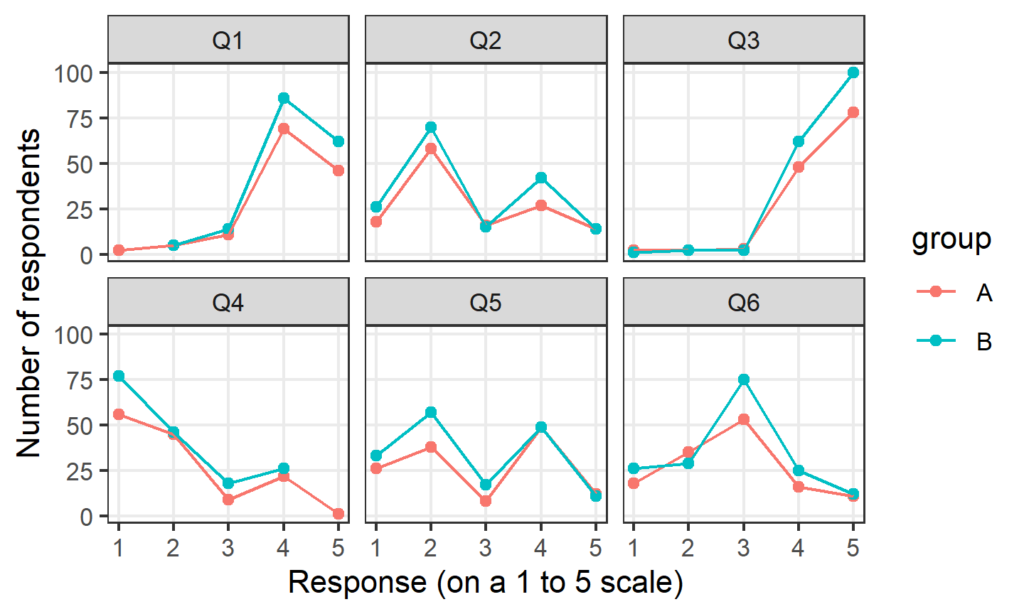
ggplot2 has a lot of functions, and many of them aren’t documented in R help pages. This post will show you how to use ggplot2’s plotting functions to make a plot. We’re going to plot a box and whisker plot of the density of cities on the planet Earth. We’re also going to show a histogram of the map data to highlight some regional differences. One thing a lot of R users ask me about is how to make plots with ggplot2, a great package for making data visualizations, especially for data that is too big to fit in a browser window. There are just so many options: different visualizations, different ways of drawing the data to the plot, and different ways to adjust the appearance. In this post, I’ll go over some of the most common functions, and how to use them.
How do you plot a function in R?
In this blog post, I will demonstrate how to use the ggplot2 package for making plots in R. This is a very basic example, but hopefully the idea will become clear. The last few weeks have been a whirlwind for me, and I haven’t had much time to write about my work in R. This is going to be my first post that is strictly about R, so I want to make sure that everyone has a good understanding of what ggplot2 is. In fact, I’m going to assume that you have never used R before.
How do you make a scatterplot in ggplot2?
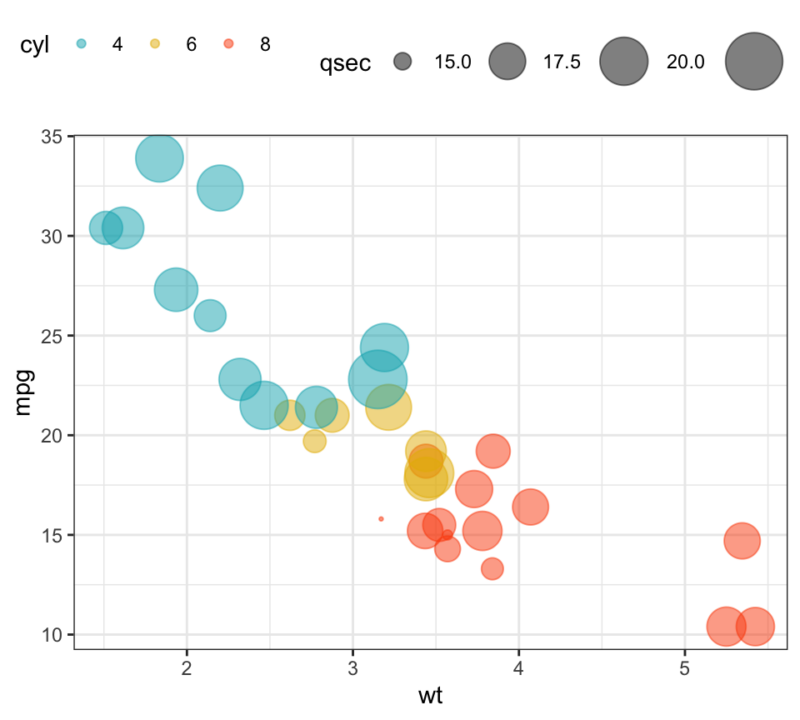
I was poking around the ggplot2 package the other day when I wondered how to make a scatter plot. In this post, I’ll explain how to make a scatter plot, and a little bit about the R programming language in general. Scatterplots are a great way to visualize data. You can look at many different ways to visualize data with ggplot2. To get started, first find some data. A good starting point is the iris dataset from the tidyverse. If you’d like to learn more, check out this blog post.
Related Tags:
ggplot2 functions in rggplot inside function not workingplot() function in rcould not find function “ggplot”creating plots in r using ggplot2ggplot add function line,People also search for,Feedback,Privacy settings,How Search works,ggplot2 functions in r,ggplot inside function not working,plot() function in r,could not find function “ggplot”,creating plots in r using ggplot2,ggplot add function line,ggplot r cheat sheet,how to use ggplot2 in rstudio